About the course curriculum
The CLA: Programming Essentials in C course (short form: C Essentials) covers the basics of programming in the C programming language and touches on fundamental programming techniques, customs and vocabulary, including the most common library functions and the usage of the pre-processor.
The aim of the course is to familiarize the student with the basic concepts of computer programming and developer tools, present the syntax, semantics and data types offered by the language, and allow the student to write his or her own programs using standard language infrastructure, regardless of the hardware or software platform.
The course is broken down into eight modules preceded by an additional Module 0, introducing the recommended programming environments, and instructions on how to install and use them.
Each student has access to hands-on practice materials, labs, quizzes, and assessments to learn how to utilize the skills and knowledge gained on the course and interact with some real-life programming tasks and situations.
Students who complete the course will be able to accomplish coding tasks related to the basics of programming in the C language, and to understand and utilize the fundamental notions and techniques used in the language.
Furthermore, they will be ready to attempt the following qualifications:
- CLE – C Certified Entry-Level Programmer (CLE-10-0x)
- CLA – C Certified Associate Programmer (CLA-11-0x).
from the C++ Institute.
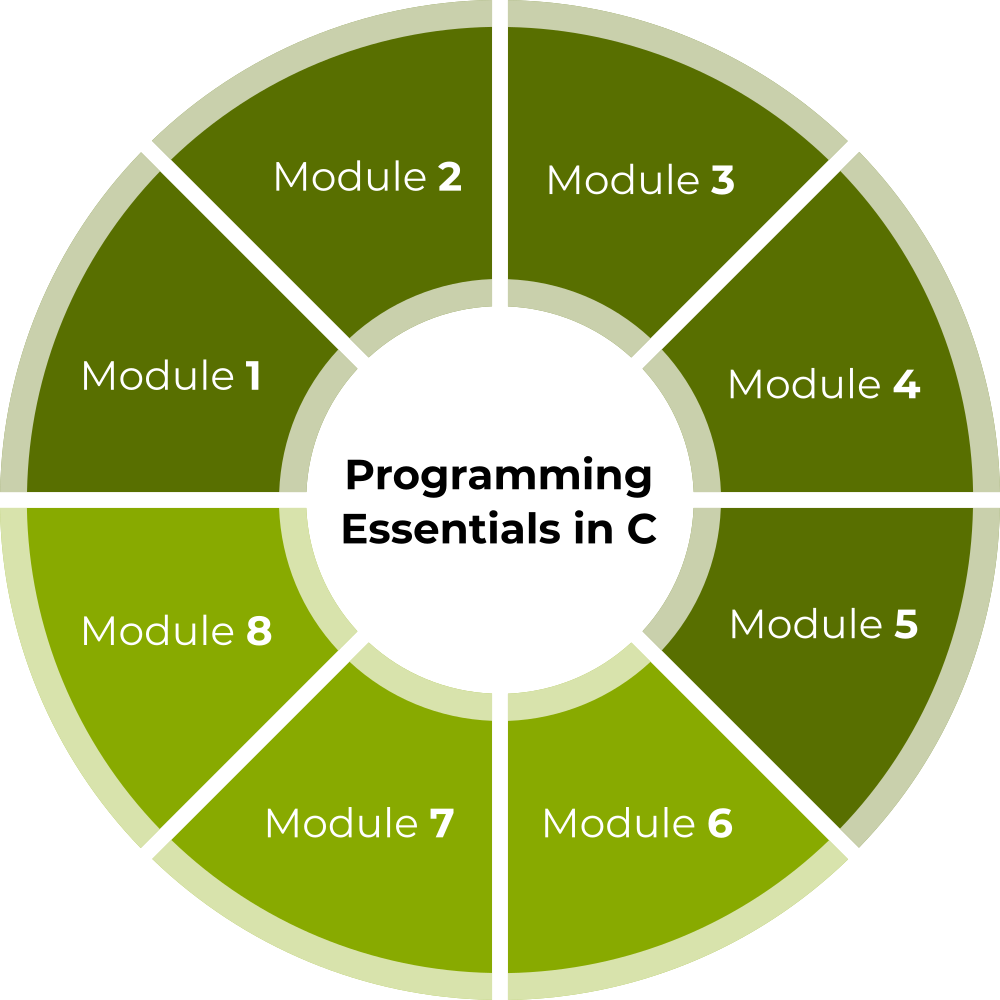
Course Modules vs. CLE and CLA Certifications (click to enlarge)
Certifications
C Essentials – Part 1 is aligned with the CLE – C Certified Entry-Level Programmer certification:
CLE – C Certified Entry-Level Programmer certification: a professional credential that measures your ability to accomplish coding tasks related to the essentials of programming in the C language. A test candidate should demonstrate sufficient knowledge of the universal concepts of computer programming, the syntax and semantics of the C language, including: data types, flow control, arrays and pointers, memory management and structure concepts, as well as demonstrate the knowledge of fundamental programming techniques characteristic of the C language, and the use of the most basic standard library functions.
CLE – C Certified Entry-Level Programmer certification shows that the individual is familiar with universal computer programming concepts like compilation, variables, data types, typecasting, operators, conditional execution, loops, arrays, pointers, structures, and the runtime environment.
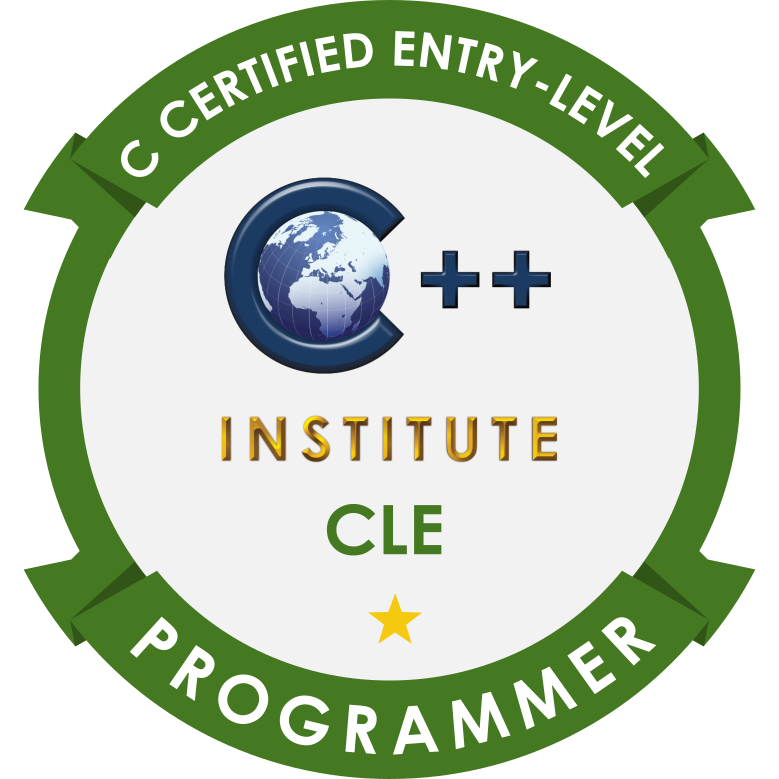
CLE – C Certified Entry-Level Programmer
C Essentials – Part 2 is aligned with the CLA – C Certified Associate Programmer certification:
CLA – C Certified Associate Programmer certification: a professional credential that measures your ability to accomplish coding tasks related to the basics of programming in the C programming language, as well as fundamental programming techniques, customs and vocabulary, including the most common library functions and the usage of the preprocessor.
CLA – C Certified Associate Programmer certification shows that the individual is familiar with all the exam scope covered by the CLE certification, as well as understanding and using such concepts as functions, files, streams, preprocessor, and complex declarations.
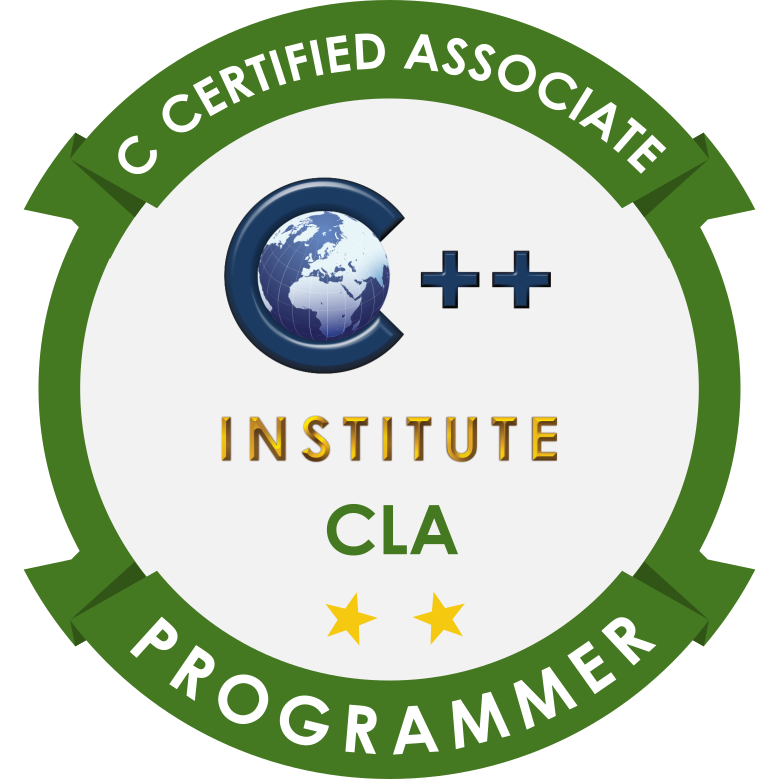
CLA – C Certified Associate Programmer
CLE – C Certified Entry-Level Programmer certification is an interim step to the CLA – C Certified Associate Programmer certification and the starting point to launch a career in software development, low-level and middle-level programming, C programming, and related technologies. Becoming CLE certified will help you stand out from other candidates and get your foot in the door.
Course syllabus
CE1: C Essentials – Part 1
Module 0: Installing And Using Your Programming Environment
- Introduction to IDE
- Online tools
- Edube Interactive
Module 1: Absolute Basics - Introduction to Computer Programming
- languages: natural and artificial,
- machine languages,
- high-level programming languages,
- obtaining the machine code: compilation process,
- writing simple programs,
- variables,
- integer values in real life and in C,
- integer literals,
- comments.
Module 2: Data Types, operations, and the basics of flow control
- floating-point values in real life and in C,
- float literals,
- arithmetic operators,
- priority and binding,
- post- and pre-incrementation, decrementation,
- operators of type op=,
- the char type and ASCII code,
- char literals,
- equivalence of int and char data,
- comparison operators,
- conditional execution and if keyword,
- the printf() and scanf() functions
Module 3: Flow Control (continued), more data types, computer logic
- conditional execution: the else branch,
- integer and float types,
- conversions,
- typecast and its operators,
- loops: while, do, and for,
- controlling the loop execution: break and continue,
- logical and bitwise operators.
Module 4: Aggregating Data Into Arrays
- switch: different faces of ‘if’,
- arrays (vectors),
- sorting in real life and computer memory,
- initiators,
- pointers,
- an address, a reference, a dereference and the sizeof operator,
- simple pointers, pointers to nothing (NULL),
- the & operator,
- pointer arithmetic,
- pointers vs. arrays: different forms of the same phenomenon,
- strings,
- string manipulation.
Module 5: Memory Management And Structures
- array indexing,
- using pointers: perils and disadvantages,
- the void type,
- arrays of arrays and multidimensional arrays,
- memory allocation and deallocation: the malloc() and free() functions,
- arrays of pointers vs. multidimensional arrays,
- structures,
- declaring, using and initializing structures,
- pointers to structures and arrays of structures,
- the basics of recursive data collections.
End of Part 1
- CE1: Part 1 Summary Test (Score 70% or more to unlock Part 2.)
CE2: C Essentials – Part 2
Module 6: Functions
- functions,
- how to declare, define and invoke functions,
- the scope of variables, local variables and function parameters,
- pointers, arrays and structures as function parameters,
- function result and the return statement,
- void as a parameter, pointer and result,
- parameterizing the main function,
- external functions and the extern declarator,
- header files and their role.
Module 7: Connecting to the Real World - Files And Streams
- files vs. streams,
- header files needed for stream operations,
- the FILE structure,
- opening and closing streams, open modes, the errno variable,
- reading and writing to/from a stream,
- predefined streams: stdin, stdout, and stderr,
- stream manipulation: the fgetc(), fputc(), fgets(), and fputs() functions,
- raw input/output: the fread(), and fwrite() functions.
Module 8: Preprocessor And Complex Declarations
- preprocessor,
- #include - how to make use of a header file,
- #define - simple and parameterized macros,
- the #undef directive,
- predefined preprocessor symbols,
- macrooperators: # and ##,
- conditional compilation: the #if and #ifdef directives,
- avoiding multiple compilations of the same header files,
- scopes of declarations, storage classes,
- user-defined types,
- pointers to functions,
- analyzing and creating complex declarations.
End of Part 2
- CE2: Part 2 Summary Test (Score 70% or more to unlock the Final Test)
- C Essentials - Final Test (Score 70% or more to be eligible for the CLA exam discount code.)