About the course curriculum
The CPA: Programming Essentials in C++ course (short form: C++ Essentials) covers the basics of programming in the C++ programming language, touches on fundamental programming techniques, customs and vocabulary, including the most common library functions and the usage of the pre-processor, as well as introduces the student to the object-oriented approach.
The aim of the course is to familiarize the student with the basic concepts of computer programming and developer tools, present the syntax, semantics and data types offered by the language, and allow the student to write his or her own programs using standard language infrastructure, regardless of the hardware or software platform.
The course is broken down into eight modules preceded by an additional Module 0, introducing the recommended programming environments, and instructions on how to install and use them.
Each student has access to hands-on practice materials, labs, quizzes, and assessments to learn how to utilize the skills and knowledge gained on the course and interact with some real-life programming tasks and situations.
Students who complete the course will be able to accomplish coding tasks related to the basics of programming in the C++ language, and to understand and utilize the fundamental notions and techniques used in the language.
Furthermore, they will be ready to attempt the following qualifications:
- CPE - C++ Certified Entry-Level Programmer (CPE-20-0x | Coming January 2021)
- CPA - C++ Certified Associate Programmer (CPA-21-0x).
from the C++ Institute.
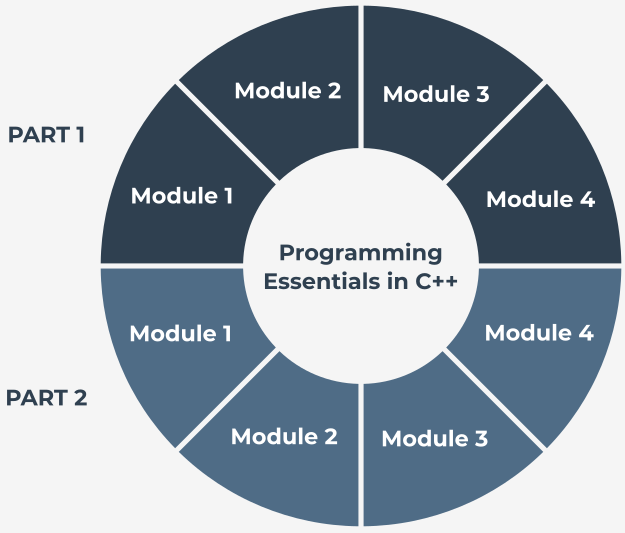
Course Modules vs. CPE and CPA Certifications (click to enlarge)
Certifications
C++ Essentials - Part 1 is aligned with the CPE - C++ Certified Entry-Level Programmer certification:
CPE - C++ Certified Entry-Level Programmer certification: a professional credential that measures your ability to accomplish coding tasks related to the essentials of programming in the C++ language. A test candidate should demonstrate sufficient knowledge of the universal concepts of computer programming, the syntax and semantics of the C language, including: data types, flow control, arrays and pointers, memory management and structure concepts, as well as demonstrate the knowledge of fundamental programming techniques characteristic of the C++ language, and the use of the most basic standard library functions.
CPE - C Certified Entry-Level Programmer certification shows that the individual is familiar with universal computer programming concepts like compilation, variables, data types, typecasting, operators, conditional execution, loops, arrays, pointers, structures, and the runtime environment.
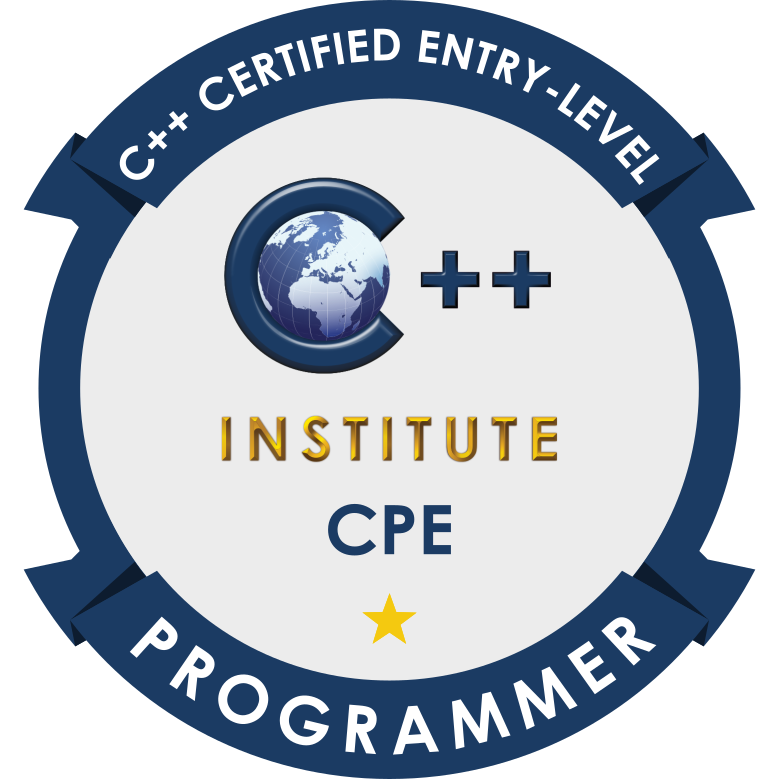
CPE - C++ Certified Entry-Level Programmer
C++ Essentials - Part 2 is aligned with the CPA - C++ Certified Associate Programmer certification:
CPA - C++ Certified Associate Programmer certification: a professional credential that measures your ability to accomplish coding tasks related to the basics of programming in the C++ programming language, as well as fundamental programming techniques, customs and vocabulary, including the most common library functions, the usage of the preprocessor, and the object-oriented approach.
CPA - C++ Certified Associate Programmer certification shows that the individual is familiar with all the exam scope covered by the CPE certification, as well as understanding and using such concepts as functions, files, streams, preprocessor, complex declarations, and the concept of object-oriented programming in C++.
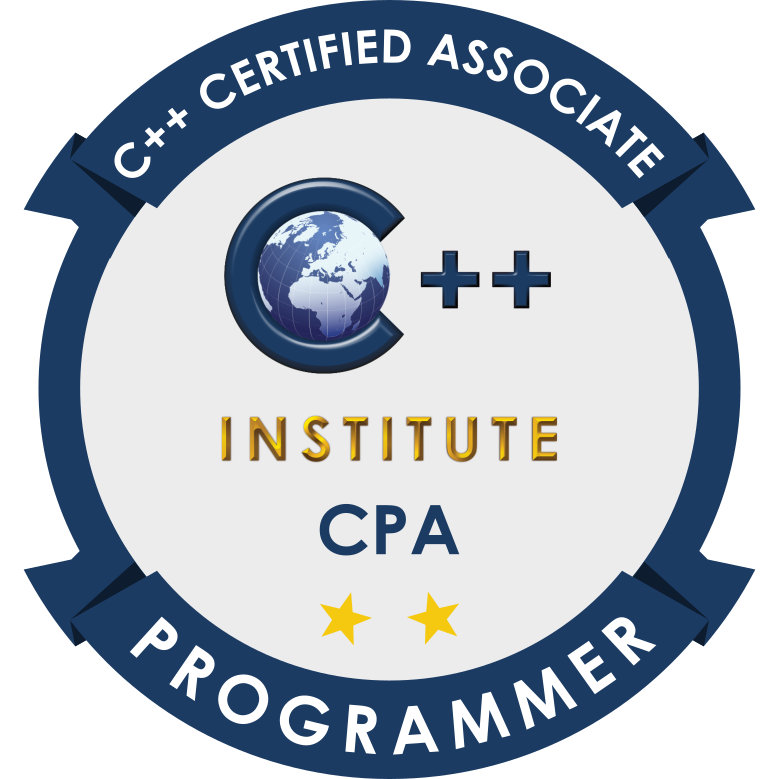
CPA - C++ Certified Associate Programmer
CPE - C++ Certified Entry-Level Programmer certification is an interim step to the CPA - C++ Certified Associate Programmer certification and the starting point to launch a career in software development, low-level, middle-level, and high-level programming, C++ programming, and related technologies. Becoming CPE certified will help you stand out from other candidates and get your foot in the door.
Course syllabus
C++ Essentials 1 (CPPE1)
Module 0: Installing And Using Your Programming Environment
- Introduction to IDE;
- Online tools;
- Edube Interactive.
CPPE1 - Module 1: Absolute Basics - Introduction to Computer Programming
- languages: natural and artificial;
- machine languages;
- high-level programming languages,
- obtaining the machine code: compilation process;
- variables;
- integer values in real life and in C++;
- integer literals;
- characters;
- comments;
- the basics of flow control;
- dealing with streams and basic I/O operations;
- writing simple programs.
CPPE1 - Module 2: Advanced flow control and data aggregates
- how to control the flow of the program;
- more data types;
- conditional instructions: if, else, switch;
- loops and controlling the loop execution;
- logic, bitwise and arithmetic operators;
- vectors, multidimensional arrays;
- declaring and initializing structures.
CPPE1 - Module 3: Extending expressive power: pointers, functions, and memory
- designing, declaring, and invoking functions;
- pointers;
- different methods of passing parameters and their purpose;
- default parameters;
- inline functions;
- overloaded functions;
- sorting;
- memory on demand.
CPPE1 - Module 4: Accessing various data types
- arrays of pointers;
- conversions;
- strings: declarations, initializations, assignments;
- strings as an example of objects: (methods and properties)
- using and declaring namespaces;
- dealing with exceptions.
CPPE1 - End of Part 1
- CPPE1: Part 1 Summary Test (Score 70% or more to unlock Part 2.)
C++ Essentials 2 (CPPE2)
CPPE2 - Module 1: The essentials of Object-Oriented Programming
- Basic concepts of OOP;
- A stack: the procedural approach vs. the OOP approach;
- The anatomy of the class;
- Static components;
- Objects vs. pointers and objects inside objects.
CPPE2 - Module 2: Inheritance
- Class hierarchies;
- Classes, inheritance, and type compatibility;
- Polymorphism and virtual methods;
- Objects as parameters, and dynamic casting;
- Various supplements;
- The const keyword;
- Friendship in the C++ world.
CPPE2 - Module 3: Exceptions
- Introduction to exceptions;
- The throw statement;
- Categorizing exceptions;
- Catching exceptions;
- Exceptions in action.
CPPE2 - Module 4: Operators and enumerated types
- Overloading operators;
- Enumerated types.
CPPE2 - End of Part 2
- CPPE2: Part 2 Summary Test (Score 70% or more to unlock the Final Test)
- C++ Essentials - Final Test (Score 70% or more to be eligible for the CPA exam discount code.)