Duck typing is a fancy name for the term describing an application of the duck test: "If it walks like a duck and it quacks like a duck, then it must be a duck", which determines whether an object can be used for a particular purpose. An object's suitability is determined by the presence of certain attributes, rather than by the type of the object itself.
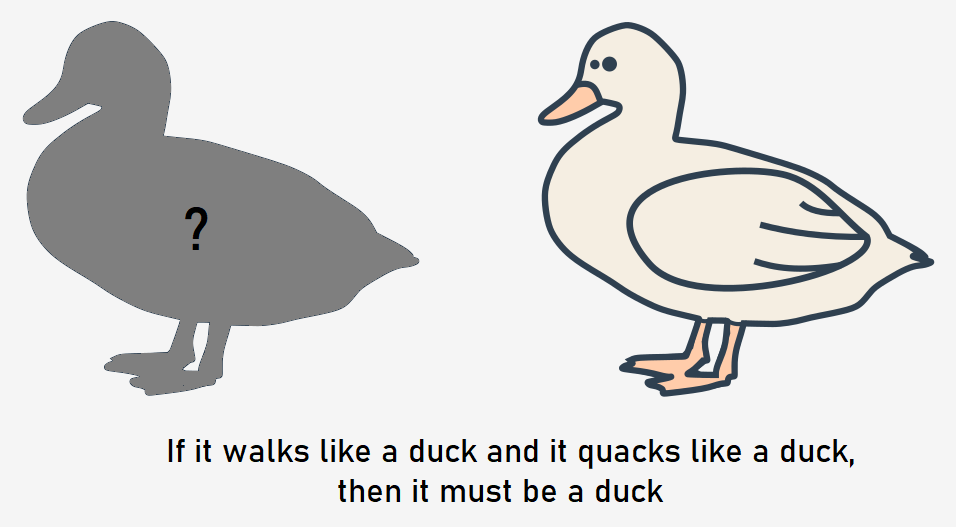
Duck typing is another way of achieving polymorphism, and represents a more general approach than polymorphism achieved by inheritance. When we talk about inheritance, all subclasses are equipped with methods named the same way as the methods present in the superclass.
In duck typing, we believe that objects own the methods that are called. If they do not own them, then we should be prepared to handle exceptions.
Let's talk about two things that share conceptually similar methods, but represent totally different things, like cheese and wax. Both can melt, and we use the melted forms for different purposes.
When you run the code you should receive the following output:
Wax can be used to form a tool
Cheese can be eaten
No melt() method
output
Both the Wax and Cheese classes own melt()
methods, but there is no relation between the two. Thanks to duck typing, we can call the melt()
method. Unfortunatelly, the Wood class is not equipped with this method, so an AttributeError exception occurs.
Code
class Wax:def melt(self):
print("Wax can be used to form a tool")
class Cheese:
def melt(self):
print("Cheese can be eaten")
class Wood:
def fire(self):
print("A fire has been started!")
for element in Wax(), Cheese(), Wood():
try:
element.melt()
except AttributeError:
print('No melt() method')