When called, functions and operators that state the core syntax are translated into magic methods delivered by specific classes.
So Python knows what to do when the interpreter spots the '+' operator between two strings or two integers, or even two Person class objects – it looks for the magic method responsible for the called function or operator in order to apply it to the operands (objects).
Of course, the '+' operator is just a handy example, and later on we'll discuss other operators.
In Python, these magic methods are sometimes called special purpose methods, because these methods are designated by design to handle specific operations.
The name of each magic method is surrounded by double underscores (Pythonistas would say “dunder” for double underscores, as it’s a shorter and more convenient phrase). Dunders indicate that such methods are not called directly, but called in a process of expression evaluation, according to Python core syntax rules.
The '+' operator is in fact converted to the __add__() method and the len() function is converted to the __len__() method. These methods must be delivered by a class (now it’s clear why we treat classes as blueprints) to perform the appropriate action.
Look at the following simple code:
number = 10
print(number + 20)
It is in fact translated to:
number = 10
print(number.__add__(20))
Try running these snippets on your own to compare the results for floats and strings.
What Python does is it executes the magic method __add__() on the left operand (“number” object in the example) of the expression, and the right operand (number 20) is passed as a method argument.
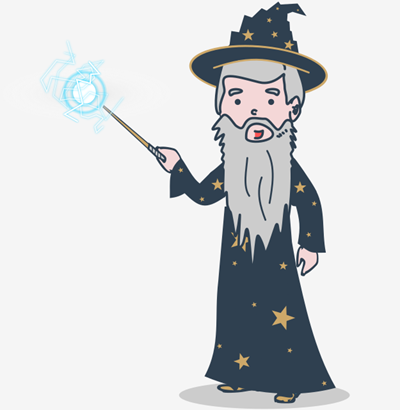