Let's pickle our first set of data consisting of:
- a nested dictionary carrying some information about currencies;
- a list containing a string, an integer, and a list.
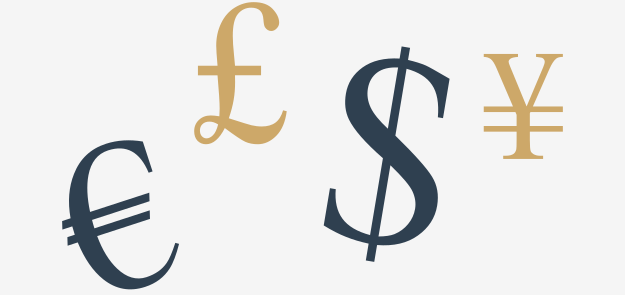
When you run the code presented in the right pane, a new file should be created. Remember to run the code locally.
The code starts with the import statement responsible for loading the pickle module:
import pickle
Later you can see that the file handle 'file_out' is associated with the file opened for writing in binary mode. It’s important to open the file in binary mode as we are dumping data as a stream of bytes.
with open('multidata.pckl', 'wb') as file_out:
Now it’s time to persist the first object with the dump()
function. This function expects an object to be persisted and a file handle.
pickle.dump(a_dict, file_out)
And the second object is persisted in the same way:
pickle.dump(a_list, file_out)
In this result, we have created a file that retains the pickled objects.
Code
import picklea_dict = dict()
a_dict['EUR'] = {'code':'Euro', 'symbol': '€'}
a_dict['GBP'] = {'code':'Pounds sterling', 'symbol': '£'}
a_dict['USD'] = {'code':'US dollar', 'symbol': '$'}
a_dict['JPY'] = {'code':'Japanese yen', 'symbol': '¥'}
a_list = ['a', 123, [10, 100, 1000]]
with open('multidata.pckl', 'wb') as file_out:
pickle.dump(a_dict, file_out)
pickle.dump(a_list, file_out)
{{ dockerServerErrorMsg }}
×
{{ errorMsg }}
×
{{ successMsg }}
×