In Python's approach, everything is an object, and every object has some type associated with it. To get the type of any object, make use of the type()
function.
Run the code in the right pane to see the type()
function in action.
<class 'int'>
<class 'list'>
<class '__main__.Dog'>
<class 'type'>
output
We can see that objects in Python are defined by their inherent classes.
The example also shows that we can create our own classes, and those classes will be instances of the type special class, which is the default metaclass responsible for creating classes.
Let's perform one more experiment that will respond to the question: what type of objects are built-in classes and the metaclass type
?
for t in (int, list, type):
print(type(t))
The results are quite interesting:
These observations lead us to the following conclusions:
- metaclasses are used to create classes;
- classes are used to create objects;
- the type of the metaclass
type
istype
– no, that is not a typo.
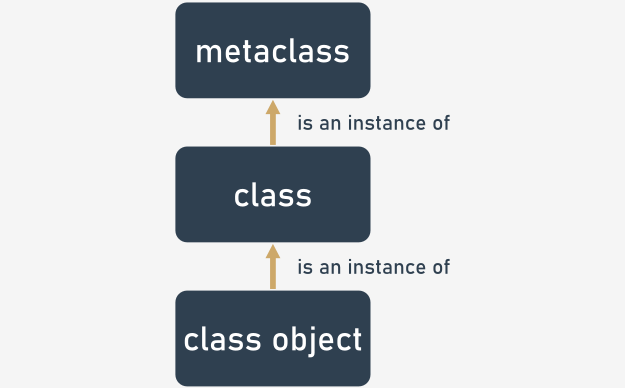
To extend the above observations, it’s important to add:
type
is a class that generates classes defined by a programmer;- metaclasses are subclasses of the
type
class.
Before we start creating our own metaclasses, it’s important to understand some more details regarding classes and the process of creating them.